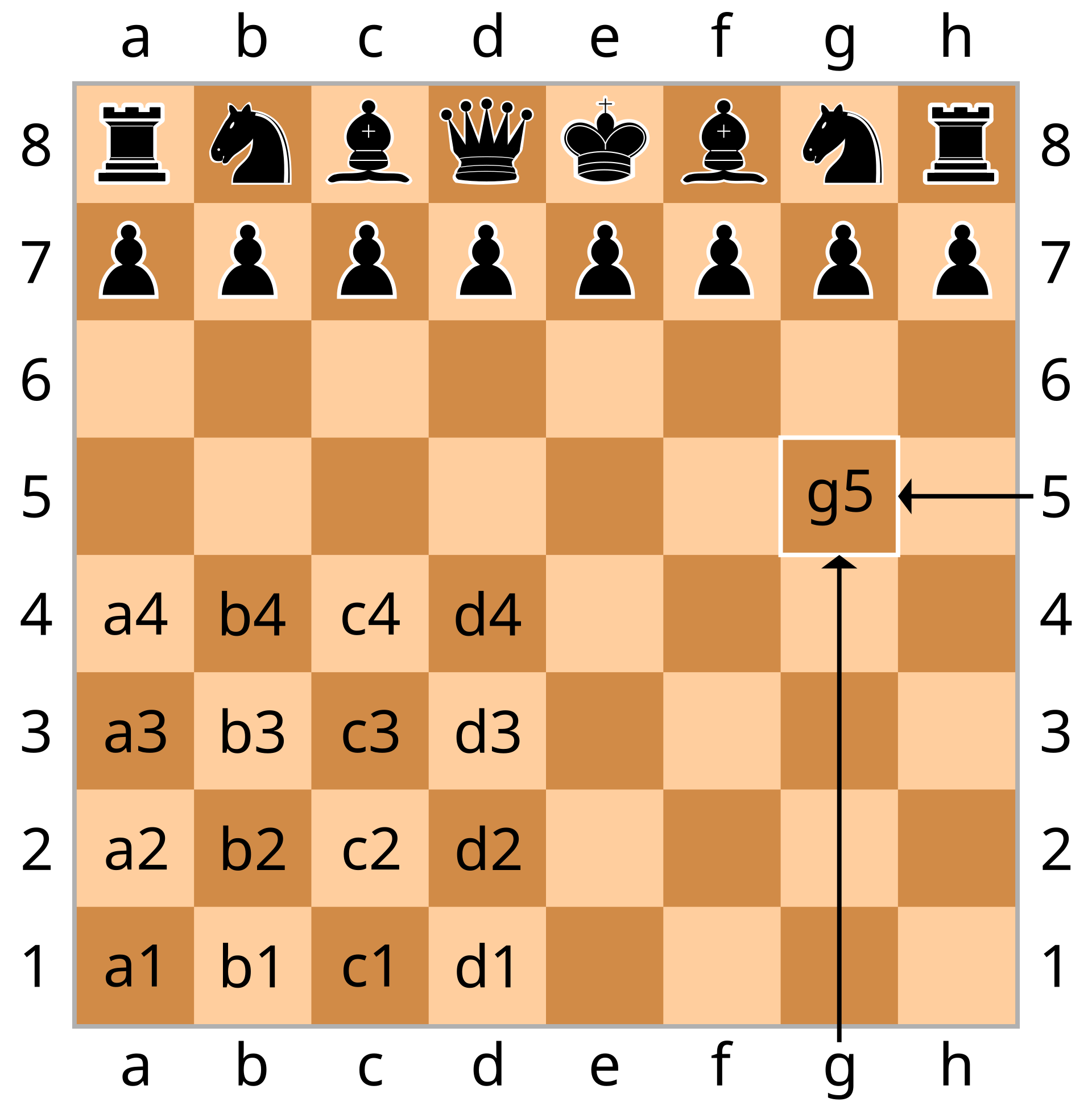
Write Python modules that form the foundation of a chess game.
Write Python modules that form the foundation of a chess game.
Prerequisites
-
function definition
-
string manipulation
-
modules
-
lists or dicts
Background
The game of chess is played on an $8 \times 8$ board. As shown in Figure 1, individual squares on this board can be named by a combination of rank (number from 1 to 8) and file (letter from a to h).
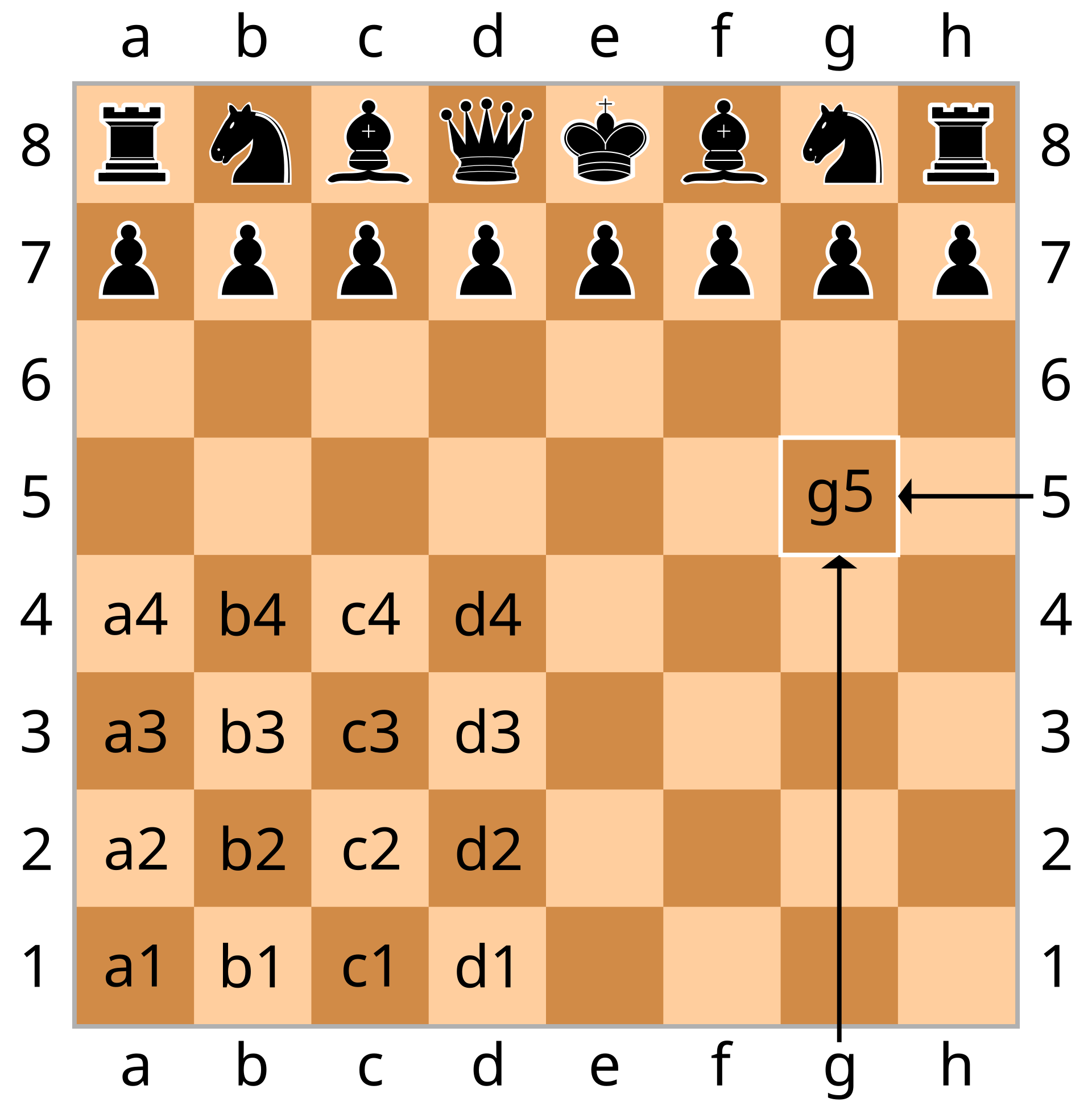
When a new game of chess is started, the pieces owned by the two players (called White and Black for the colours of their pieces) are placed in defined starting positions:
Square | Player | Piece |
---|---|---|
a1 |
White |
Rook |
b1 |
White |
Knight |
c1 |
White |
Bishop |
d1 |
White |
Queen |
e1 |
White |
King |
f1 |
White |
Bishop |
g1 |
White |
Knight |
h1 |
White |
Rook |
a2 |
White |
Pawn |
b2 |
White |
Pawn |
c2 |
White |
Pawn |
d2 |
White |
Pawn |
e2 |
White |
Pawn |
f2 |
White |
Pawn |
g2 |
White |
Pawn |
h2 |
White |
Pawn |
a7 |
Black |
Pawn |
b7 |
Black |
Pawn |
c7 |
Black |
Pawn |
d7 |
Black |
Pawn |
e7 |
Black |
Pawn |
f7 |
Black |
Pawn |
g7 |
Black |
Pawn |
h7 |
Black |
Pawn |
a8 |
Black |
Rook |
b8 |
Black |
Knight |
c8 |
Black |
Bishop |
d8 |
Black |
Queen |
e8 |
Black |
King |
f8 |
Black |
Bishop |
g8 |
Black |
Knight |
h8 |
Black |
Rook |
Objective
Your objective in this assignment is write two Python modules that will work together to form the foundation of a chess game. These modules should be implemented as described below.
board
module
This module should enable the creation, modification and inspection of a chess board. It should contain the following functions:
new_board()
-
This function should accept no arguments and return an empty board. That board can be represented however you like (list, array, other data type), as long as it is accepted by the other functions described below.
colour(board, square)
-
Returns the colour of piece that is found on a given square. For example, at the beginning of a game,
colour(board, "a1")
should return "W" for White. If the square is empty, returnsNone
. count_pieces(board, colour)
-
Returns the number of pieces on a board owned by a specified player (
"B"
for Black,"W"
for White). piece(board, square)
-
Returns the letter representing the type of piece occupying a square:
Piece | Result |
---|---|
King |
|
Queen |
|
Rook |
|
Bishop |
|
Knight |
|
Pawn |
|
Nothing (empty) |
|
place_piece(board, square, colour, piece)
-
Places a piece of a specified colour (player) and kind (e.g.,
N
for knight) onto the board at the specified square. pretty(board)
-
This function should return a string that nicely represents ("pretty-prints") a board in a way that people can interpret. It should return a string and not print anything. There is no specified requirement for how the board should look. An example of the kind of thing you might consider doing (again, not required) would be:
a b c d e f g h 8 □ □ □ □ □ □ □ □ 8 7 □ □ □ □ □ □ □ □ 7 6 □ □ □ □ □ □ □ □ 6 5 □ □ □ □ □ □ □ □ 5 4 □ □ □ □ □ □ □ □ 4 3 □ □ □ □ □ □ □ □ 3 2 □ □ □ □ □ □ □ □ 2 1 □ □ □ □ □ □ □ □ 1 a b c d e f g h
chess
module
new_game()
-
This function should create a new game with Black’s and White’s pieces in their starting positions. The function should return a tuple of the board and the current turn. To start with, it should be White’s turn, so the returned
turn
value should be"W"
. print_game_state(b, turn)
-
Given a board and the current turn, print out (using
print()
) a summary of the current game state. For example, ifturn == "B"
, you might print "it is Black’s turn" followed by a human-readable printout of the board.
Procedure
I would suggest starting by creating the required Python modules as empty
Python files and submitting them.
Then, add stub functions that do nothing but pass
or return None
.
Then, finally, work to implement the various functions.
You should test your work and then submit to Gradescope. Remember, assignments are individual work: you must complete the assignment yourself.
Sample tests
Although I’d encourage you to write and share your own tests, here is a simple test program that should get you started:
Save this file in the same directory as your board.py
and chess.py
and then run it to see how your code performs.